Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Hardware/mapping.getUsb
Explore with Pulumi AI
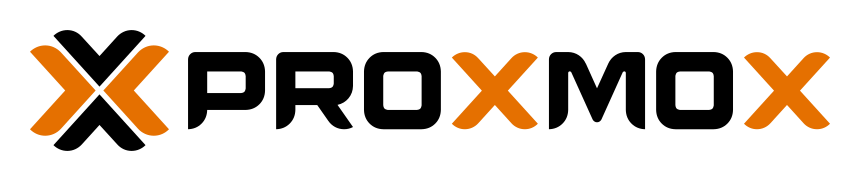
Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves a USB hardware mapping from a Proxmox VE cluster.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const example = proxmoxve.Hardware.mapping.getUsb({
name: "example",
});
export const dataProxmoxVirtualEnvironmentHardwareMappingUsb = example;
import pulumi
import pulumi_proxmoxve as proxmoxve
example = proxmoxve.Hardware.mapping.get_usb(name="example")
pulumi.export("dataProxmoxVirtualEnvironmentHardwareMappingUsb", example)
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Hardware"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
example, err := Hardware.GetUsb(ctx, &mapping.GetUsbArgs{
Name: "example",
}, nil)
if err != nil {
return err
}
ctx.Export("dataProxmoxVirtualEnvironmentHardwareMappingUsb", example)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example = ProxmoxVE.Hardware.Mapping.GetUsb.Invoke(new()
{
Name = "example",
});
return new Dictionary<string, object?>
{
["dataProxmoxVirtualEnvironmentHardwareMappingUsb"] = example,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Hardware_mapping.Hardware_mappingFunctions;
import com.pulumi.proxmoxve.Hardware.inputs.GetUsbArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var example = Hardware/mappingFunctions.getUsb(GetUsbArgs.builder()
.name("example")
.build());
ctx.export("dataProxmoxVirtualEnvironmentHardwareMappingUsb", example.applyValue(getUsbResult -> getUsbResult));
}
}
variables:
example:
fn::invoke:
Function: proxmoxve:Hardware/mapping:getUsb
Arguments:
name: example
outputs:
dataProxmoxVirtualEnvironmentHardwareMappingUsb: ${example}
Using getUsb
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getUsb(args: GetUsbArgs, opts?: InvokeOptions): Promise<GetUsbResult>
function getUsbOutput(args: GetUsbOutputArgs, opts?: InvokeOptions): Output<GetUsbResult>
def get_usb(name: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetUsbResult
def get_usb_output(name: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetUsbResult]
func GetUsb(ctx *Context, args *GetUsbArgs, opts ...InvokeOption) (*GetUsbResult, error)
func GetUsbOutput(ctx *Context, args *GetUsbOutputArgs, opts ...InvokeOption) GetUsbResultOutput
> Note: This function is named GetUsb
in the Go SDK.
public static class GetUsb
{
public static Task<GetUsbResult> InvokeAsync(GetUsbArgs args, InvokeOptions? opts = null)
public static Output<GetUsbResult> Invoke(GetUsbInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetUsbResult> getUsb(GetUsbArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:Hardware/mapping/getUsb:getUsb
arguments:
# arguments dictionary
The following arguments are supported:
- Name string
- The name of this USB hardware mapping.
- Name string
- The name of this USB hardware mapping.
- name String
- The name of this USB hardware mapping.
- name string
- The name of this USB hardware mapping.
- name str
- The name of this USB hardware mapping.
- name String
- The name of this USB hardware mapping.
getUsb Result
The following output properties are available:
- Comment string
- The comment of this USB hardware mapping.
- Id string
- The unique identifier of this USB hardware mapping data source.
- Maps
List<Pulumi.
Proxmox VE. Hardware. Mapping. Outputs. Get Usb Map> - The actual map of devices for the hardware mapping.
- Name string
- The name of this USB hardware mapping.
- Comment string
- The comment of this USB hardware mapping.
- Id string
- The unique identifier of this USB hardware mapping data source.
- Maps
[]Get
Usb Map - The actual map of devices for the hardware mapping.
- Name string
- The name of this USB hardware mapping.
- comment String
- The comment of this USB hardware mapping.
- id String
- The unique identifier of this USB hardware mapping data source.
- maps
List<Get
Usb Map> - The actual map of devices for the hardware mapping.
- name String
- The name of this USB hardware mapping.
- comment string
- The comment of this USB hardware mapping.
- id string
- The unique identifier of this USB hardware mapping data source.
- maps
Get
Usb Map[] - The actual map of devices for the hardware mapping.
- name string
- The name of this USB hardware mapping.
- comment str
- The comment of this USB hardware mapping.
- id str
- The unique identifier of this USB hardware mapping data source.
- maps
Sequence[hardware.mapping.
Get Usb Map] - The actual map of devices for the hardware mapping.
- name str
- The name of this USB hardware mapping.
- comment String
- The comment of this USB hardware mapping.
- id String
- The unique identifier of this USB hardware mapping data source.
- maps List<Property Map>
- The actual map of devices for the hardware mapping.
- name String
- The name of this USB hardware mapping.
Supporting Types
GetUsbMap
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
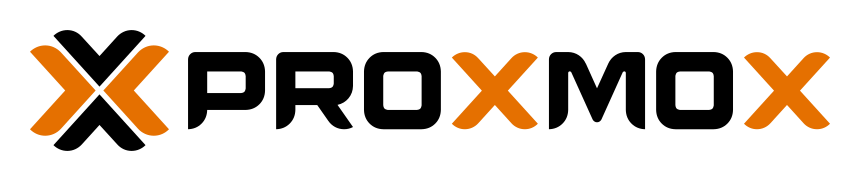
Proxmox Virtual Environment (Proxmox VE) v6.10.1 published on Friday, Jun 28, 2024 by Daniel Muehlbachler-Pietrzykowski